This REST API for the Clinic Management System is developed using the Laravel framework. It provides essential CRUD operations and includes key features such as doctor management, patient records, patient tests, patient appointments, billing, and payments. The API enables the creation, updating, and deletion of doctor and patient records while supporting appointment scheduling, health assessments, and financial transactions. Additionally, it ensures secure user authentication and role-based access control for administrators and staff, with API security enforced through token-based authentication.
Sample POST APIs
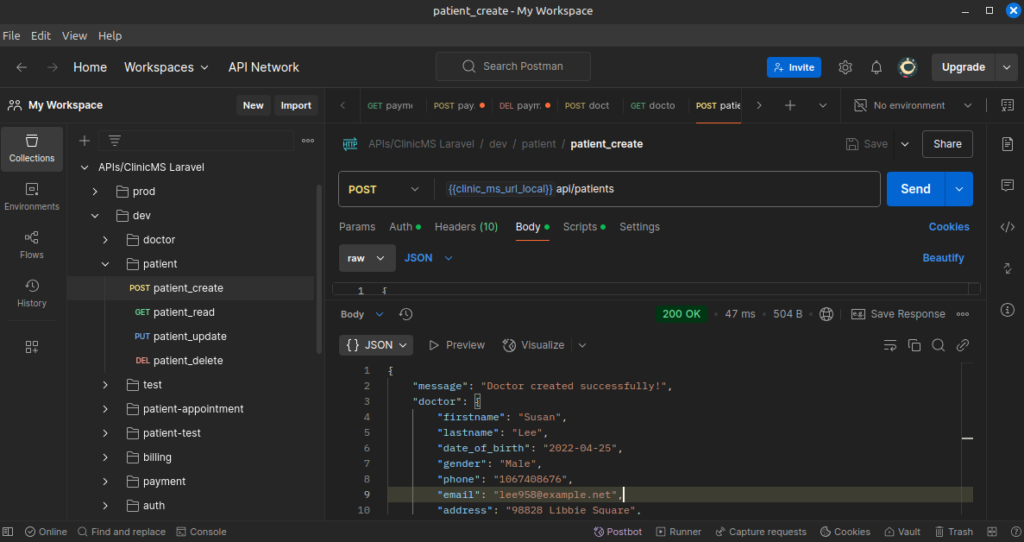
Doctor’s API
POST
curl --location 'http://jdjportolio.com/laravel/clinic-ms-api/doctors' \
--header 'Content-Type: application/json' \
--header 'Authorization: ••••••' \
--data '{
"firstname": "Jose",
"lastname": "Kwelyo",
"specialty": "Surgeon",
"phone": "+1-479-251-0648",
"email": "[email protected]"
}'
Sample Result:
{
"message": "Doctor created successfully!",
"doctor": {
"firstname": "Frank",
"lastname": "Nelson",
"specialty": "Pediatrician",
"phone": "7815222875",
"email": "[email protected]",
"id": 101
}
}
Patient’s API
POST
curl --location 'http://jdjportolio.com/laravel/clinic-ms-api/patients' \
--header 'Content-Type: application/json' \
--header 'Authorization: ••••••' \
--data '{
"firstname": "Susan",
"lastname": "Lee",
"date_of_birth": "2022-04-25",
"gender": "Female",
"phone": "1067408676",
"email": "[email protected]",
"address": "98828 Libbie Square",
"city": "Port Dion",
"area": "Market Square",
"zipcode": "PK"
}'
Sample Result:
{
"message": "Patient created successfully!",
"doctor": {
"firstname": "Susan",
"lastname": "Lee",
"date_of_birth": "2022-04-25",
"gender": "Female",
"phone": "1067408676",
"email": "[email protected]",
"address": "98828 Libbie Square",
"city": "Port Dion",
"area": "Market Square",
"zipcode": "PK",
"id": 601
}
}
Patient Examination API
POST
curl --location 'http://jdjportolio.com/laravel/clinic-ms-api/patient-tests' \
--header 'Content-Type: application/json' \
--header 'Authorization: ••••••' \
--data '{
"patient_id": 50,
"test_id": 2,
"doctor_id": 300,
"appointment_id": 1,
"test_date": "2025-03-29 00:00:01",
"status": "pending",
"result": "test result"
}'
Sample Result:
{
"message": "Patient Test created successfully!",
"patient_test": {
"appointment_test_id": null,
"patient_id": 50,
"test_id": 2,
"doctor_id": 300,
"appointment_id": 1,
"test_date": "2025-03-29 00:00:01",
"status": "pending",
"result": "test result"
}
}
Patient Appointment API
POST
curl --location 'http://jdjportolio.com/laravel/clinic-ms-api/appointments' \
--header 'Content-Type: application/json' \
--header 'Authorization: ••••••' \
--data '{
"patient_id": 104,
"doctor_id": 339,
"appointment_date": "2025-03-29",
"status": "scheduled"
}'
Sample Result:
{
"message": "Apointment created successfully!",
"doctor": {
"patient_id": 104,
"doctor_id": 339,
"appointment_date": "2025-03-29",
"status": null
}
}
Billing API
POST
curl --location 'http://jdjportolio.com/laravel/clinic-ms-api/billings' \
--header 'Content-Type: application/json' \
--header 'Authorization: ••••••' \
--data '{
"patient_id": 55,
"appointment_id": 3,
"billing_date": "2025-03-29 10:00:01",
"total_amount": 5604,
"payment_status": "insurance",
"notes": "test note"
}'
Sample Result:
{
"message": "Billing created successfully!",
"billing": {
"billing_id": null,
"patient_id": 55,
"appointment_id": 3,
"billing_date": "2025-03-29 10:00:01",
"total_amount": 5604,
"payment_status": "insurance",
"notes": "test note"
}
}
Payment API
POST
curl --location 'http://jdjportolio.com/laravel/clinic-ms-api/payments' \
--header 'Content-Type: application/json' \
--header 'Authorization: ••••••' \
--data '{
"patient_id": 60,
"amount": 3500,
"payment_method": "credit card",
"status": "pending"
}'
Sample Result:
{
"message": "Payment record created successfully",
"payment": {
"patient_id": 60,
"amount": 3500,
"payment_method": "credit card",
"status": "pending"
}
}
Role: Sole Developer
The basic source code is available in my public GitLab repository: View Git Project